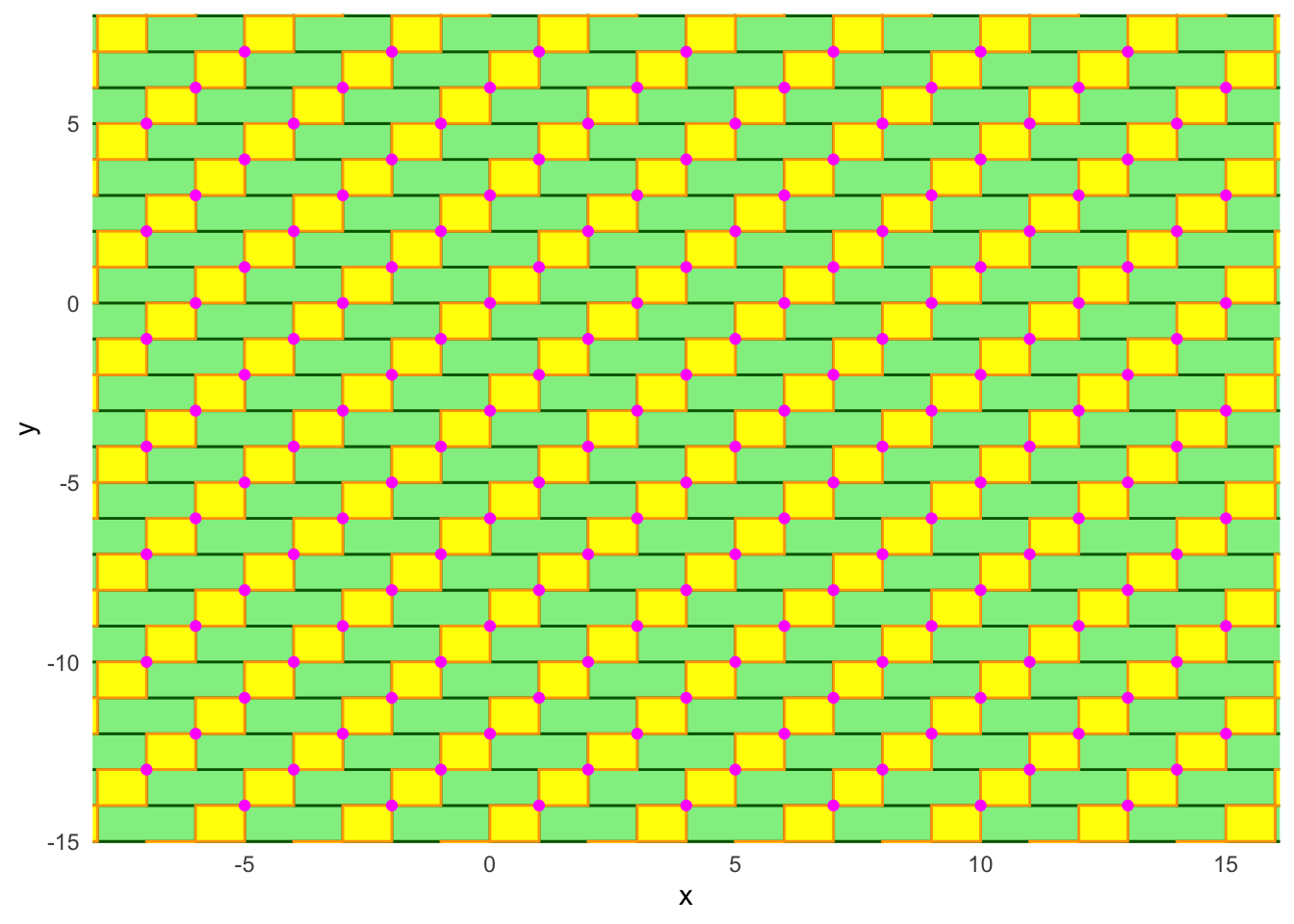
Julia’s First Tiles
Hand-drawn
First Tile
\[ M = \begin{bmatrix}2 & 1\\ -1 & 1\end{bmatrix} \]
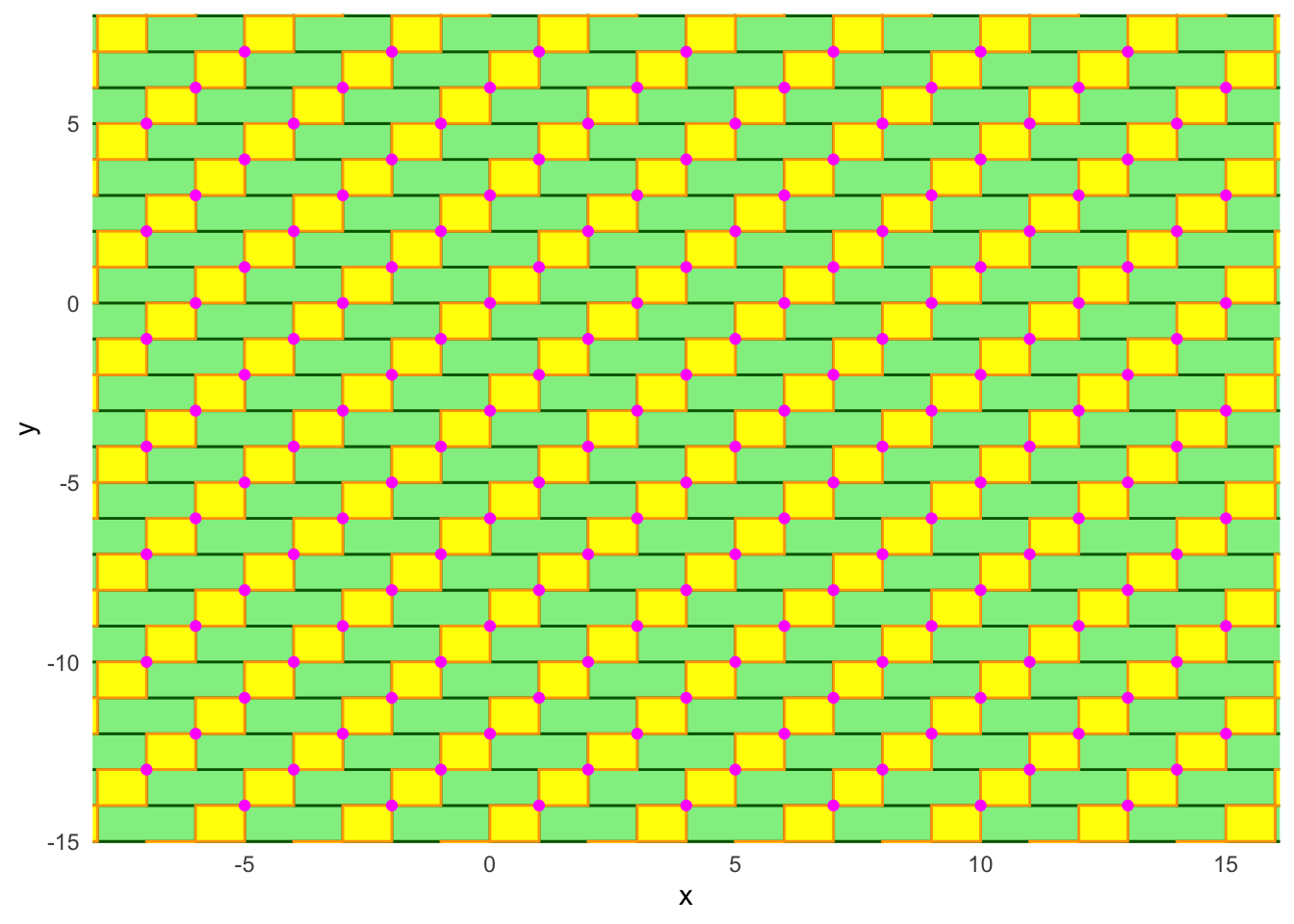
Second Tile
\[ M = \begin{bmatrix} 2 & 5\\ -1 & 3\end{bmatrix} \]
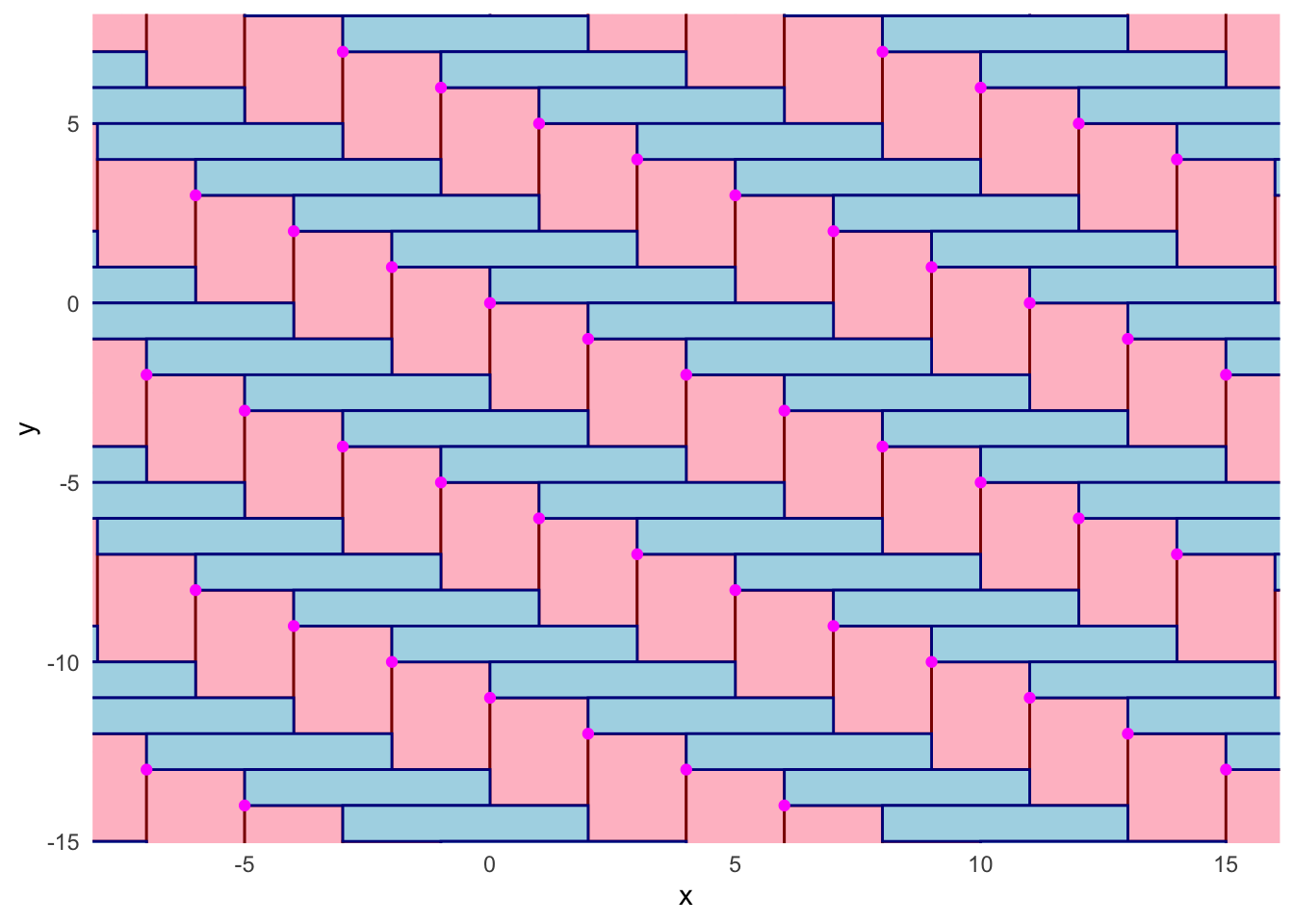
Third Tile
\[ M = \begin{bmatrix} 8& 5\\ -4 & 3\end{bmatrix} \]
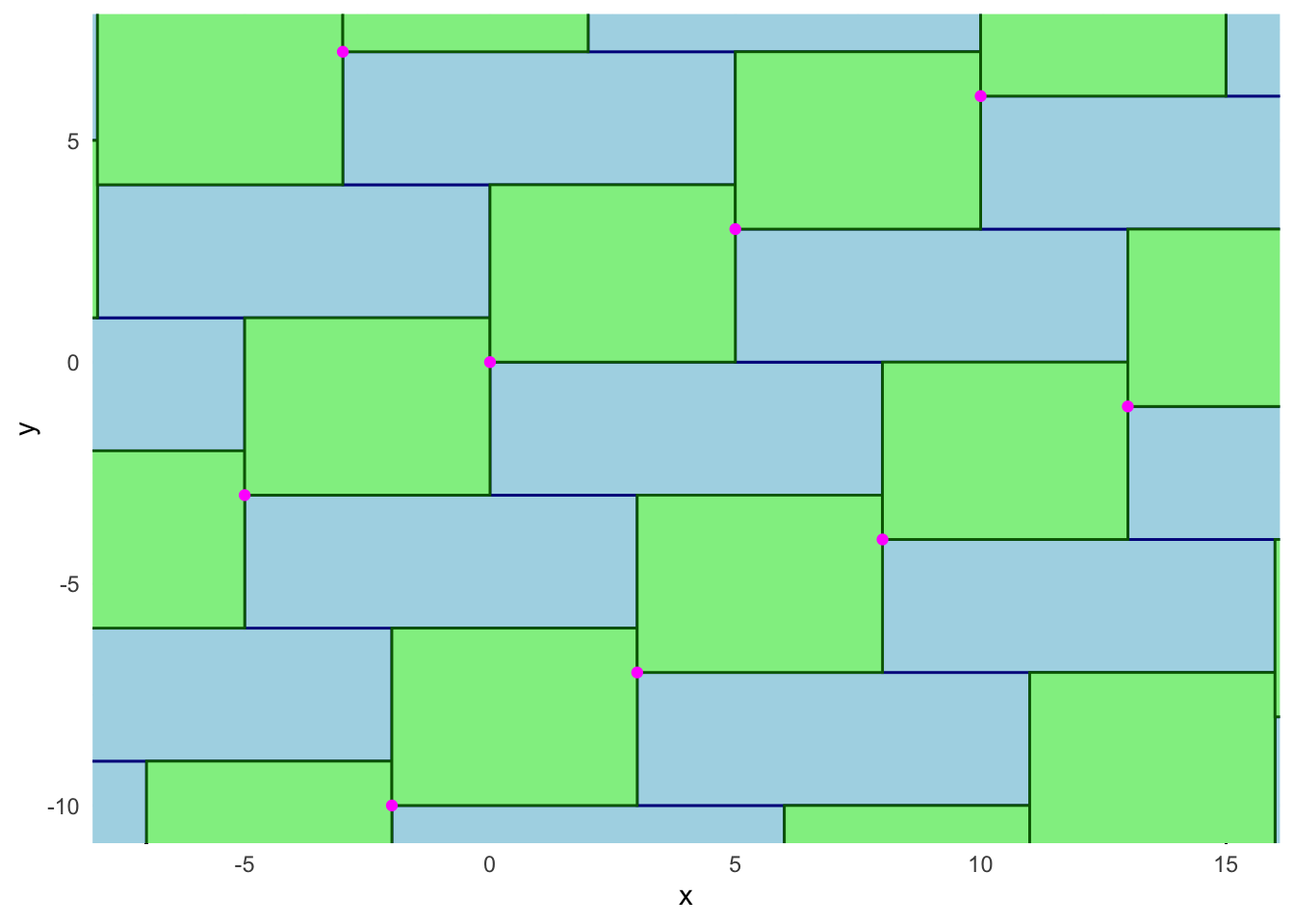
Computer-generated
Fourth Tile
\[ M = \begin{bmatrix} 3 & 3\\ -3 & 3\end{bmatrix} \]
Code
library(ggplot2)
## original coordinates
<- matrix(c(3, -3), ncol = 1)
A <- matrix(c(3, 3), ncol = 1)
B
## coordinates for 4 copies/combos
<- -10:10
copies <- expand.grid(copies, copies)
coefs
<- coefs$Var1*A[1] + coefs$Var2*B[1]
x.coords <- coefs$Var1*A[2] + coefs$Var2*B[2]
y.coords
<- data.frame(x = x.coords, y = y.coords)
plot.dat <- ggplot(data = plot.dat, aes(x = x, y = y)) +
p geom_point() +
ylim(c(-14, 7)) +
xlim(c(-7, 15)) +
geom_rect(xmin = -7, xmax = 15, ymin = -14, ymax = 7,
fill = "#FFFFFF00", col = "black") +
theme_minimal()
## could fix the grid to make these for printing!
## create on-diag rectangles
<- p + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
p1 ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "lightpink", col = "pink") +
ylim(c(-10, 7)) +
xlim(c(-7, 15))
## create off-diag rectangles
<- p1 + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + B[1],
p2 ymin = plot.dat$y, ymax = plot.dat$y - A[2],
fill = "lavender", col = "purple")
+ geom_point(col = "magenta") p2
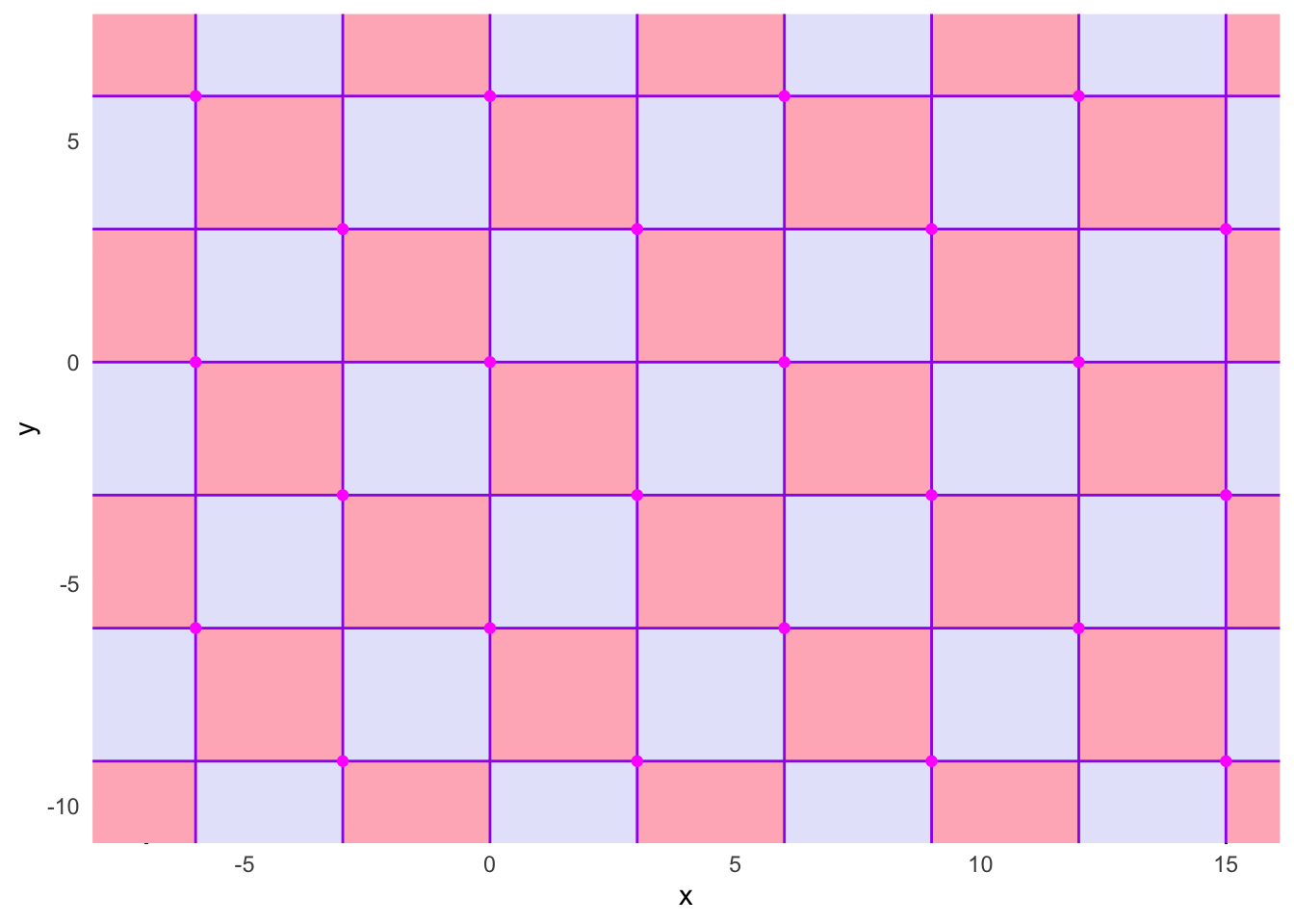
Fifth Tile
\[ M = \begin{bmatrix} 12& 6\\ -3 & 1\end{bmatrix} \]
Code
library(ggplot2)
## original coordinates
<- matrix(c(12, -3), ncol = 1)
A <- matrix(c(6, 1), ncol = 1)
B
## coordinates for 4 copies/combos
<- -10:10
copies <- expand.grid(copies, copies)
coefs
<- coefs$Var1*A[1] + coefs$Var2*B[1]
x.coords <- coefs$Var1*A[2] + coefs$Var2*B[2]
y.coords
<- data.frame(x = x.coords, y = y.coords)
plot.dat <- ggplot(data = plot.dat, aes(x = x, y = y)) +
p geom_point() +
ylim(c(-14, 7)) +
xlim(c(-7, 15)) +
geom_rect(xmin = -7, xmax = 15, ymin = -14, ymax = 7,
fill = "#FFFFFF00", col = "black") +
theme_minimal()
## could fix the grid to make these for printing!
## create on-diag rectangles
<- p + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
p1 ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "lightpink", col = "darkred") +
ylim(c(-10, 7)) +
xlim(c(-7, 15))
## create off-diag rectangles
<- p1 + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + B[1],
p2 ymin = plot.dat$y, ymax = plot.dat$y - A[2],
fill = "#E0FFFF", col = "#A2FFFF")
+ geom_point(col = "magenta") p2
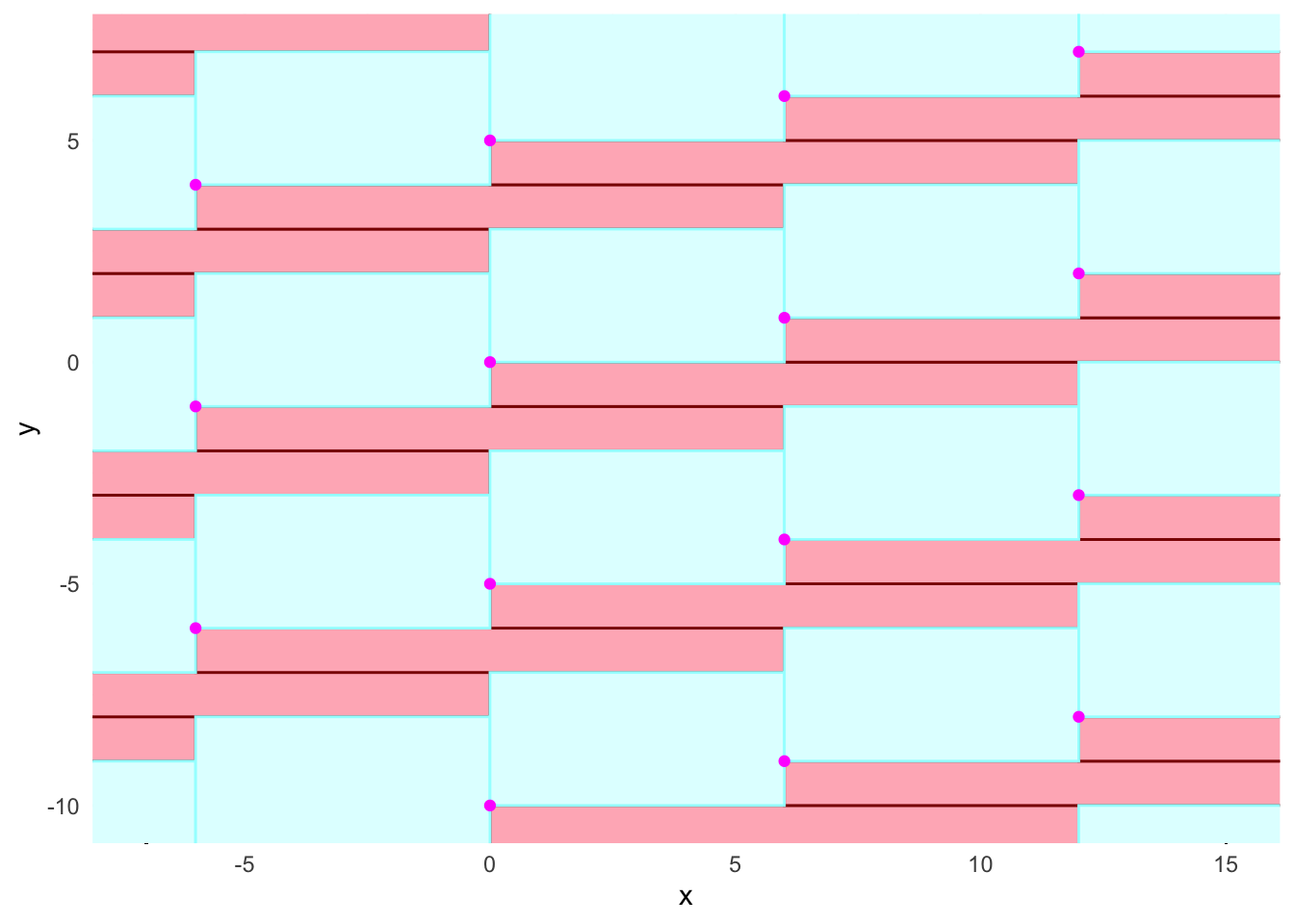
Sixth tile
Code
library(ggplot2)
## original coordinates
<- matrix(c(7, 5), ncol = 1)
A <- matrix(c(6, 2), ncol = 1)
B
## coordinates for 4 copies/combos
<- -15:15
copies <- expand.grid(copies, copies)
coefs
<- coefs$Var1*A[1] + coefs$Var2*B[1]
x.coords <- coefs$Var1*A[2] + coefs$Var2*B[2]
y.coords
<- data.frame(x = x.coords, y = y.coords)
plot.dat <- ggplot(data = plot.dat, aes(x = x, y = y)) +
p geom_point() +
ylim(c(-14, 7)) +
xlim(c(-7, 15)) +
# geom_rect(xmin = -7, xmax = 15, ymin = -14, ymax = 7,
# fill = "#FFFFFF00", col = "black") +
theme_void()
## could fix the grid to make these for printing!
## create on-diag rectangles
<- p +geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + B[1],
p1 ymin = plot.dat$y, ymax = plot.dat$y - A[2],
fill = "#008b8b", alpha = .1) +
geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + B[1],
ymin = plot.dat$y, ymax = plot.dat$y - A[2],
fill = "#FFFFFF00", col = "grey") +
geom_rect(xmin = plot.dat$x[481], xmax = plot.dat$x[481] + B[1],
ymin = plot.dat$y[481], ymax = plot.dat$y[481] - A[2],
fill = "#FFFFFF00", col = "blue") +
geom_point(col = "magenta") +
ylim(c(-14, 7)) +
xlim(c(-7, 15))
library(patchwork)
<- p + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
p2 ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "yellow", alpha = .3) +
geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "#FFFFFF00", col = "grey") +
geom_rect(xmin = plot.dat$x[481], xmax = plot.dat$x[481] + A[1],
ymin = plot.dat$y[481], ymax = plot.dat$y[481] - B[2],
fill = "#FFFFFF00", col = "orange")+
geom_point(col = "magenta")+
ylim(c(-14, 7)) +
xlim(c(-7, 15))
## create off-diag rectangles
<- p1 + geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
p_both ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "yellow", alpha = .2) +
## outlines
geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + B[1],
ymin = plot.dat$y, ymax = plot.dat$y - A[2],
fill = "#FFFFFF00", col = "lightblue", lwd = 2) +
geom_rect(xmin = plot.dat$x, xmax = plot.dat$x + A[1],
ymin = plot.dat$y, ymax = plot.dat$y - B[2],
fill = "#FFFFFF00", col = "lightyellow") +
geom_rect(xmin = plot.dat$x[481], xmax = plot.dat$x[481] + B[1],
ymin = plot.dat$y[481], ymax = plot.dat$y[481] - A[2],
fill = "#FFFFFF00", col = "blue", lwd = 2) +
geom_rect(xmin = plot.dat$x[481], xmax = plot.dat$x[481] + A[1],
ymin = plot.dat$y[481], ymax = plot.dat$y[481] - B[2],
fill = "#FFFFFF00", col = "yellow") +
geom_point(col = "magenta") +
ylim(c(-14, 7)) +
xlim(c(-7, 15))
## add edges after
+ p2 + p_both p1
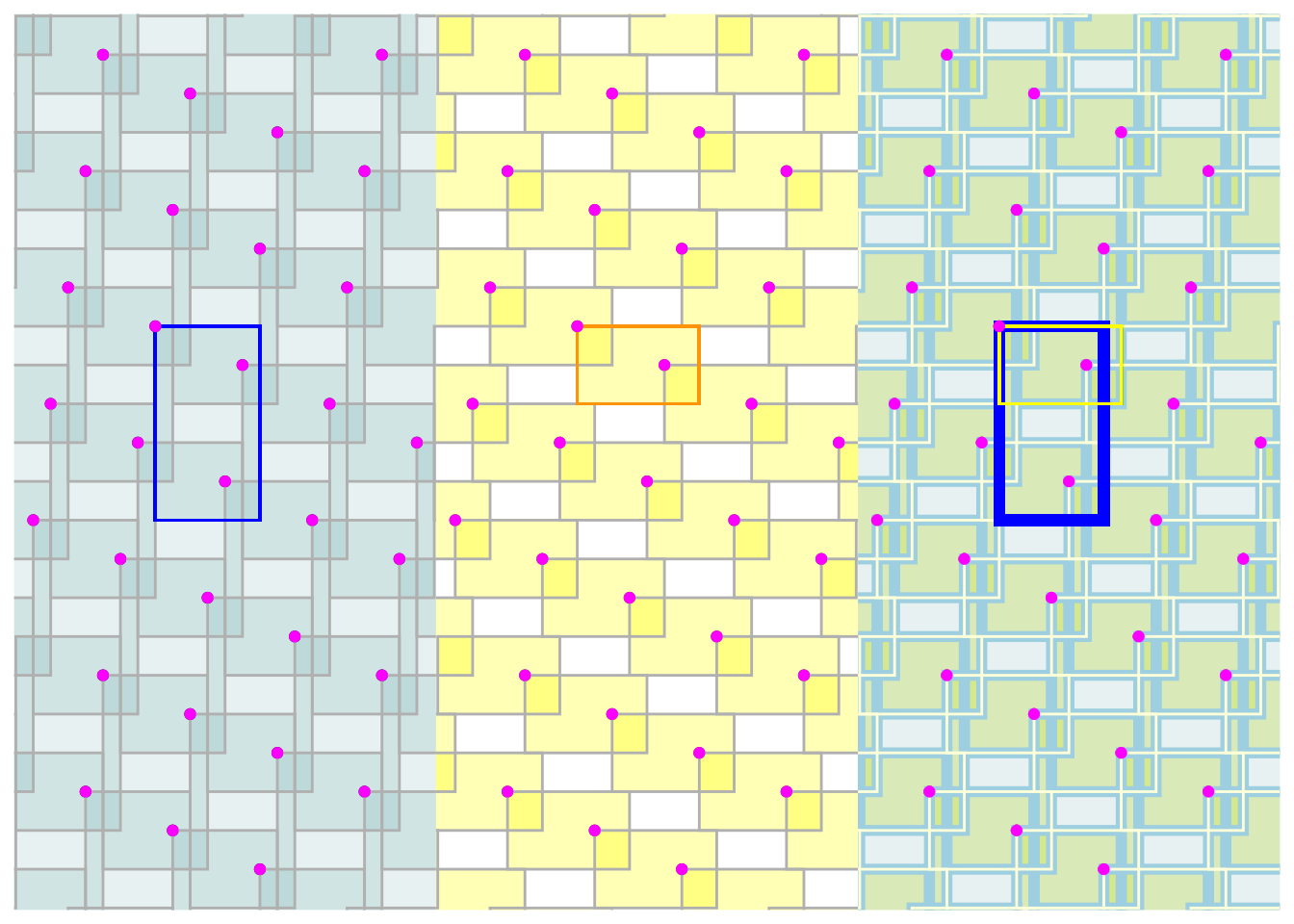